Blog
React Design Patterns
Stay informed on new product features, the latest in technology, solutions, and updates.
Sep 6, 2023
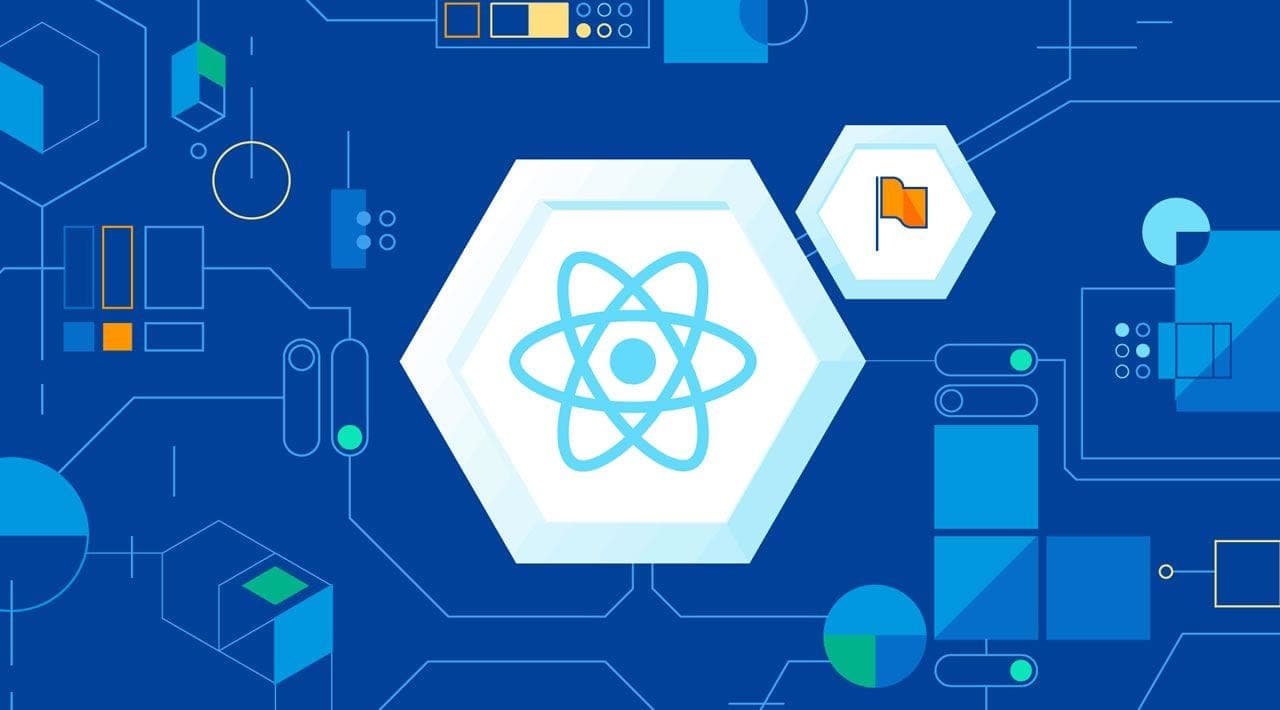
Design Patterns are the pre-built templates that help create websites and mobile applications efficiently. In simple terms, design patterns are the proven solutions to resolve common application problems and development challenges.
While the term "design patterns" might sound complex, it essentially refers to proven solutions to common coding challenges. Think of them as refined techniques discovered and refined by experienced developers. These patterns empower you to create React applications that are not only elegant but also exhibit qualities such as clarity, scalability, and resilience.
React Components design pattern
The higher-order component pattern
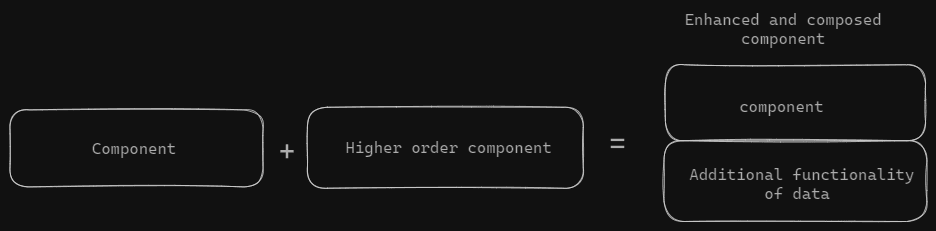
The Higher Order Component (HOC) pattern in React is a technique where a function takes a component and returns a new, enhanced component. This pattern allows for the reuse of logic across different components. Essentially, HOCs enable component composition by wrapping components with additional capabilities or modifying their behavior. It's a way to abstract and share common functionalities without directly altering the original components, helps in code modularity and reusability in React applications.
Here is an example of Higher order Component
The provider pattern
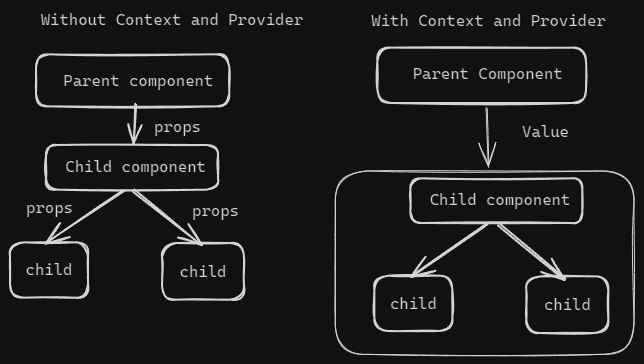
The Provider pattern in React involves using a provider component to share data or state across multiple components without having to pass it explicitly using props at each level of the component tree. It uses the Context API, enabling the creation of a central data store that components can access when needed. This helps us to maintain the states easily reducing the need for prop drilling and facilitates the management of global state within a React application.
Here is an example of Provider component
The state reducer pattern
The Reducer pattern in React involves using the useReducer hook to manage state . It has a function called a "reducer" to handle state manipulation based on dispatched actions. This pattern is particularly useful for complex state logic. The reducer takes the current state and an action as inputs and returns the new state. using this pattern,we can achieve a more maintainable and scalable approach to state management within our applications.
Here is an example of useReducer hook
Controlled inputs
A controlled element in React is an input element whose value is controlled by the state of a React component. Instead of relying solely on the browser to manage the input's state, React components manage and update the input's value through state, allowing for more predictable and controlled behavior. This is achieved by setting the input value as a state variable and providing an onChange
handler to update that state when the input changes. Controlled elements provide a centralized way to manage form data in React applications, enabling easy synchronization between the UI and component state.
Here is an example of controlled input
The hook pattern
React Hooks, a feature introduced in React 16.8, have become intergral part in the evolution of React application development. These hooks, fundamental functions, serve as gateways for functional components to harness the power of state and lifecycle methods, previously exclusive to class components. What makes hooks particularly powerful is their adaptability – they can be tailored to meet specific component requirements, offering versatility beyond their primary use cases.
mostly used hooks are useState ,useEffect ,useRef etc.
Here is an example for custom hook for api request using axios
Props Combination
Props combination is a pattern in React where related props are consolidated into a single object and passed as a unified prop to a component. This simplifies code, enhances readability, and is particularly useful when dealing with numerous related properties. By centralizing related props into a cohesive object, code becomes cleaner, more manageable, and helps reusability. It involves using object destructuring and the spread operator to efficiently handle and distribute props within components, making the codebase more organized and maintainable.
Here is an example of Props Combination
Conclusion
In conclusion, React design patterns serve as invaluable guides for developers seeking to optimize their code. These patterns, akin to seasoned strategies, enhance the structure, scalability, and overall quality of React applications. As you embark on your coding endeavors, incorporating these patterns will undoubtedly empower you to create more efficient, maintainable, and robust solutions. Happy coding!