Blog
Optimizing Node.js Applications: A Comprehensive Guide
Stay informed on new product features, the latest in technology, solutions, and updates.
Jan 24, 2024
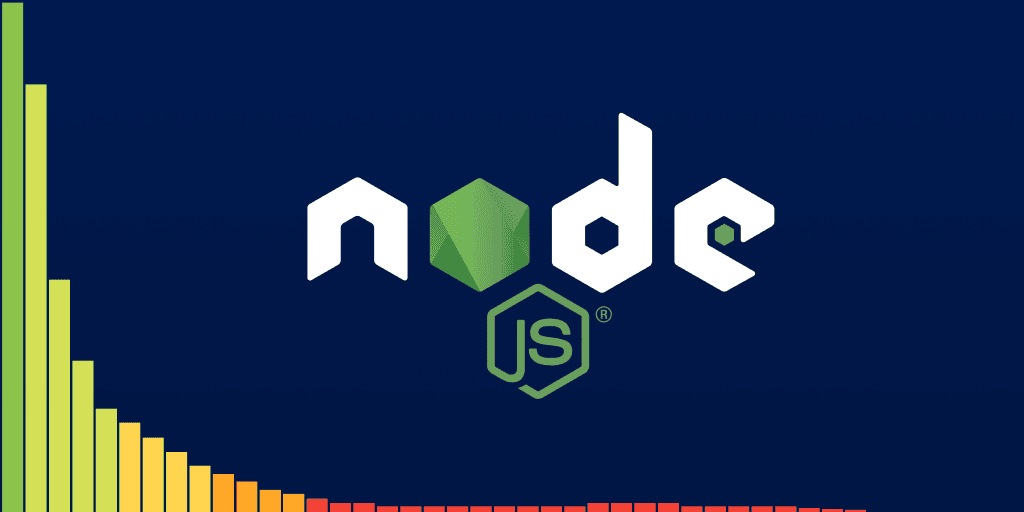
Introduction
In the realm of web development, Node.js has emerged as a powerhouse for building scalable and efficient applications. Yet, as developers, we understand that writing code that works is only half the battle. Ensuring that your Node.js application performs optimally under various loads and user interactions is critical to its success. This is where performance profiling and monitoring come into play — they are essential skills in a Node.js developer's repertoire for diagnosing issues and improving application efficiency.
Before diving into the tools and techniques for performance tuning, it's worth revisiting the Node.js architecture, particularly aspects that relate directly to performance. Node.js operates on a single-thread, event-driven non-blocking I/O model, which ideally maximizes throughput and efficiency in server-side applications. However, the very design that lends Node.js its strengths also poses unique challenges when it comes to performance bottlenecks, such as CPU-intensive operations or inefficient memory use. Understanding the internals of the Node.js event loop, the nature of asynchronous execution, and the V8 JavaScript engine's optimization strategies is pivotal in identifying and resolving performance issues.
Understanding Performance Metrics
In the context of Node.js, several key performance metrics warrant attention:
- CPU Utilization: indicates how effectively the Node.js process is using the available CPU resources. High CPU usage might be a sign of CPU-bound operations that block the event loop or computation-heavy tasks that need optimization.
- Memory Management: involves monitoring the Node.js process's memory use, which includes the heap memory managed by the V8 JavaScript engine. Memory leaks or frequent garbage collection can severely impact performance.
- Event Loop Latency: measures the responsiveness of the event loop. A saturated event loop can lead to increased latency in event handling and can be a symptom of I/O-bound problems.
- Garbage Collection: involves tracking the cycle of memory allocation and deallocation managed by the V8 engine's garbage collector. While necessary, the garbage collection process can also momentarily halt execution, thus impacting performance.
- Throughput and Response Times: refer to the number of requests a Node.js server can handle over time and the time taken to respond to these requests, respectively. These metrics are fundamental indicators of an application's capacity and responsiveness.
Profiling and monitoring are not about sporadic checks; they represent a continuous process aimed at tuning and maintaining an application's health over its lifecycle. By maintaining awareness of these metrics in real-time, a developer can quickly detect performance anomalies, identifying and rectifying them before they escalate into more significant issues.
Next, we will explore how to profile Node.js applications to gain insights into their performance behavior and what tools are available to help streamline this process.
Profiling Node.js Applications
Profiling is an analytical process to measure the performance characteristics of an application. It allows developers to identify where their Node.js application spends the most time or consumes the most resources. Profiling can highlight inefficiencies, such as CPU bottlenecks or memory leaks, and is key to understanding how to optimize your application.
What is profiling and its significance?
By methodically recording various statistics and events during an application's execution, profiling provides developers with a detailed view of the inner workings of their application under actual and simulated workloads. This allows for targeted optimizations, leading to better resource management and quicker execution times — ultimately leading to a smoother and faster user experience.
Built-in Node.js Profiler
Node.js includes a built-in profiler that can be used to perform CPU and memory profiling. This profiler is part of the V8 JavaScript engine, and while not as detailed as some third-party tools, it provides a solid starting point for profiling Node.js applications.
-inspect
flag and Chrome DevTools
Example: Using One common method for profiling Node.js apps is to start the application with the --inspect
flag, which enables the V8 inspector interface:
The above command gives you a URL that can be opened in Google Chrome. By navigating to chrome://inspect
in your browser and selecting your Node.js application from the list of available targets, you can access Chrome DevTools and start profiling.
Screenshot: Enable Profiling in Chrome DevTools
Once connected to Chrome DevTools, you can record a CPU profile by going to the "Profiler" tab and hitting the "Start" button to record your app's CPU usage. To analyze memory, head over to the "Memory" tab and take a heap snapshot or record allocation timelines.
Documentation: Node.js Debugging Guide
Profiling with clinic.js
Clinic.js is a powerful tool created to help Node.js developers find performance bottlenecks. It provides a suite of tools, including Clinic Doctor, Clinic Flame, and Clinic Bubbleprof, which give different perspectives on performance:
- Doctor: Diagnoses common Node.js performance issues
- Flame: Provides a flame graph to visualize hot code paths
- Bubbleprof: Visualizes asynchronous bottlenecks
Step-by-step guide to detect performance bottlenecks with Clinic.js
- Install Clinic.js globally on your system using npm:
- Use Clinic Doctor to start diagnosing your Node.js application by running:
Screenshot: Clinic Doctor Output Example
- For a more detailed view of your CPU activity, use Clinic Flame:
Screenshot: Clinic Flame Graph
- To understand the asynchronous operations of your applications, such as I/O, database queries, or microtasks, use Clinic Bubbleprof:
Screenshot: Clinic Bubbleprof Visualization
After running each tool, Clinic.js will generate a report that you can open in your web browser, providing visual insights into your application's performance.
Documentation: Clinic.js Official Documentation
Using these profiling tools, Node.js developers can obtain an in-depth understanding of application performance, enabling them to make informed decisions about where to focus their optimization efforts. Whether you are monitoring the CPU utilization, tracking memory allocations, or assessing the event loop's health, these tools provide the critical insights needed to boost application performance effectively.
Monitoring Tools and Integrations
Beyond profiling which is usually a one-off or periodic activity, performance monitoring is an ongoing process that ensures your Node.js application maintains optimal performance and stability during its operation. Numerous tools and services can help with this task, providing real-time insights, historical data analysis, and alerting mechanisms for any potential issues.
PM2 for Process Monitoring and Management
PM2 is a production process manager with a built-in load balancer for Node.js applications. It allows you to keep applications alive forever, reload them without downtime, and facilitate common system admin tasks.
Example: Setting up PM2 and Interpreting the Dashboard
To get started with PM2, install it globally via npm:
Then, you can start your application with PM2 like this:
Screenshot: PM2 in Action
PM2 provides a simple dashboard that shows you the status of your managed processes, CPU, and memory usage. For a more advanced monitoring experience, PM2 Plus offers a web interface that provides detailed analytics, logs, and more.
Documentation: PM2 Official Documentation
Prometheus and Grafana for Detailed Metrics
To gain deep insights into the metrics of a Node.js application, many developers turn to Prometheus and Grafana. Prometheus is an open-source monitoring system with a dimensional data model, flexible query language, and time-series data storage. Grafana is an open-source analytics and monitoring platform that integrates with Prometheus to provide rich visualization of the collected data.
Example: Configuring Prometheus and Creating Dashboards in Grafana
To monitor Node.js applications with Prometheus, you'll first need to expose metrics from the application using a client library, like prom-client
.
Install prom-client
in your Node.js application:
Expose metrics on a /metrics
endpoint:
Setting up Prometheus involves configuring a prometheus.yml
file to scrape metrics from this endpoint and storing them for Grafana to visualize.
Once your data is collected by Prometheus, you can create a dashboard in Grafana by adding Prometheus as a data source and then configuring panels to display the metrics you're interested in.
Screenshot & Guide: Using Prometheus and Grafana
Documentation: Prometheus, Grafana
Application Performance Monitoring (APM) Services
For more comprehensive performance monitoring, APM services such as New Relic, Datadog, or Dynatrace can be used to provide detailed insights and real-time analytics. These services typically offer integration with Node.js, enabling tracking of web transactions, external requests, database queries, and more.
Example: Integrating with New Relic APM
Integrating New Relic with a Node.js application starts with installing the New Relic APM agent:
You then add the newrelic
module as the first line of your application's main module to ensure the agent is loaded and able to instrument your application:
Finally, you'll configure the newrelic.js
configuration file with your license key and application details.
Screenshot & Guide: New Relic APM with Node.js
Documentation: New Relic Node.js
These are just a few examples of performance monitoring tools that can seamlessly integrate with your Node.js application. Selecting the right combination of tools and leveraging their capabilities for real-time and historical analysis is vital in maintaining a performant and reliable Node.js application. Incorporating these monitoring solutions into your DevOps practices will ensure smooth operations and allow you to address performance issues proactively.
Datadog for Real-Time Monitoring and Alerts
Datadog is a monitoring service that provides full-stack observability for modern applications, including those built on Node.js. It combines metrics, traces, and logs in one platform to provide a detailed view of an application's performance and the health of its underlying infrastructure.
Example: Integrating Node.js with Datadog
To integrate Datadog with a Node.js application, Datadog provides an npm package dd-trace
that acts as an APM agent for collecting performance data:
Initialize the tracer as early as possible in the application's setup:
After initialization, you can customize the tracer configuration to meet your application's needs, and Datadog will start collecting and visualizing the performance data in its dashboard.
Screenshot & Guide: Datadog APM Setup for Node.js
Documentation: Datadog APM
Real-world Case Studies
The true value of profiling and monitoring tools is best demonstrated through real-world scenarios and case studies where their application has resulted in tangible performance improvements or the resolution of critical issues.
Case Study 1: Resolving a Memory Leak in a Node.js Application
Memory leaks can cause an application to consume more and more memory over time, eventually leading to slow performance and crashes due to resource exhaustion. A Node.js e-commerce platform was facing such an issue where the server's memory footprint was increasing steadily under normal load.
Using heap profiling tools available in Chrome DevTools, the development team recorded heap snapshots at regular intervals when the application was running. By comparing these snapshots and analyzing the heap size growth, they identified a pattern of retained objects that were not being garbage-collected.
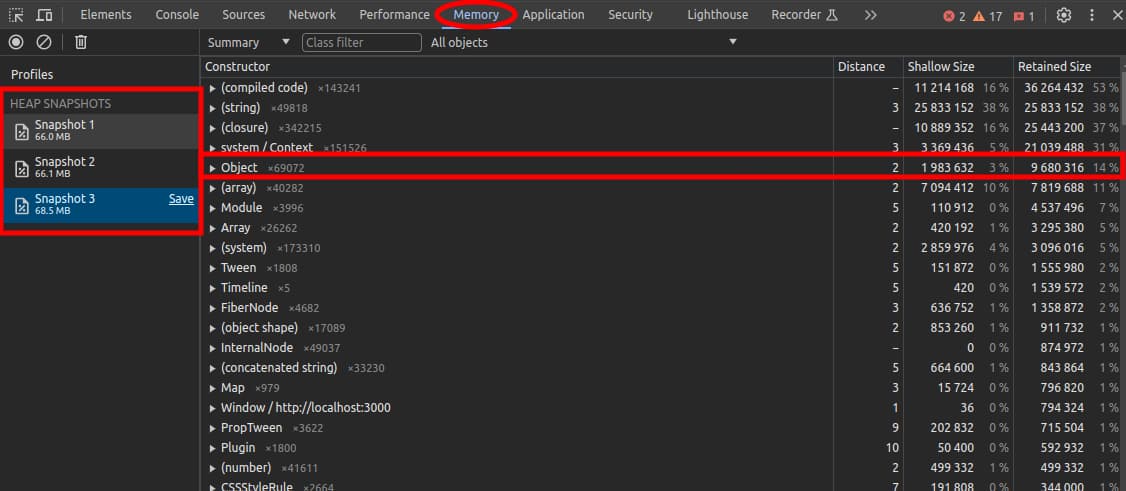
This analysis led them to discover a closure that was unintentionally holding references to large objects after the user sessions ended. The bug was resolved by updating the function to ensure that references to out-of-scope objects were properly dereferenced, allowing the garbage collector to reclaim the memory.
Documentation & Guide: Chrome DevTools Memory Analysis
Case Study 2: Optimizing CPU-Intensive Tasks with Profiling Data
A social media analytics service found that their Node.js backend was experiencing high CPU usage spikes, leading to degradation in response times during peak loads. Despite having a well-architected microservices setup, the compute-heavy task of processing large volumes of data in real-time was identified as a bottleneck.
By employing CPU profiling using clinic.js's Clinic Flame tool, the engineers were able to generate flame graphs that pinpointed the functions and calls responsible for most of the CPU computations.
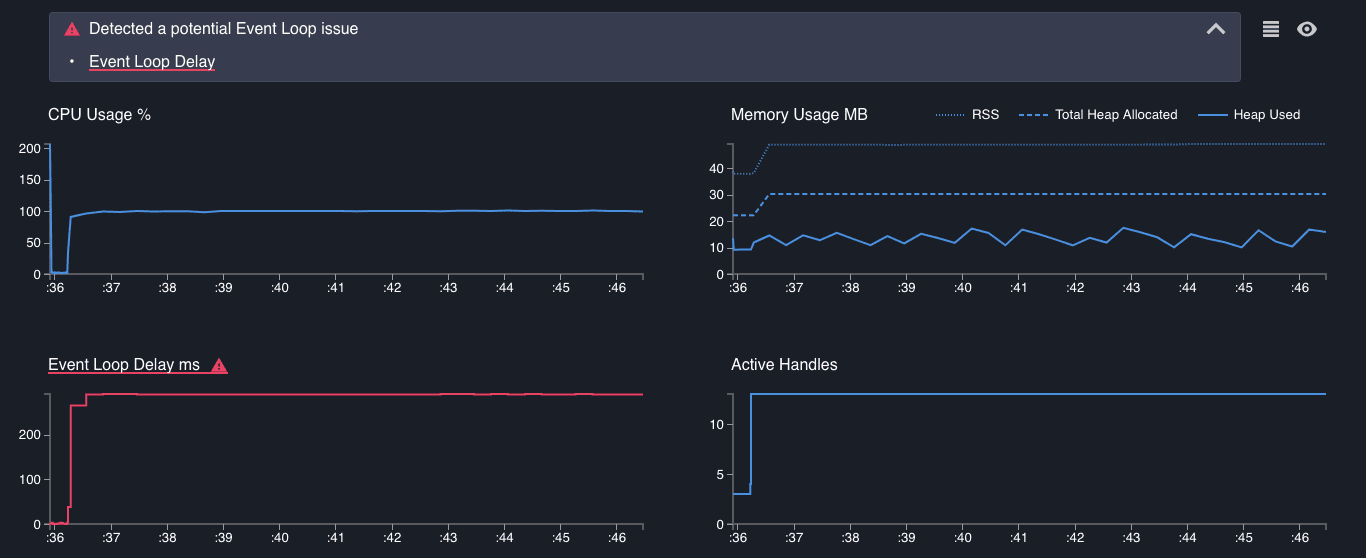
The insights from the flame graph led to a two-fold optimization approach. First, resource-intensive functions were optimized for efficiency by refactoring inefficient algorithms and eliminating unnecessary computations. Second, some of the heavy lifting was offloaded to worker threads, taking advantage of multi-threading to spread the workload across multiple CPU cores.
Documentation & Guide: Clinic.js Flame Graph
By tackling the issues using informed strategies derived from real-time profiling and monitoring data, the teams in these case studies were able to enhance application performance and resolve critical problems more efficiently than they could have through trial-and-error debugging. These case studies exemplify the importance of performance tools in maintaining and optimizing complex Node.js applications in production environments.
Advanced Performance Optimization
Once you have a baseline understanding of your Node.js application's performance through profiling and monitoring, you can dive deeper into advanced performance optimization techniques. These strategies go beyond general best practices, targeting specific areas within Node.js that can be tuned for optimal efficiency.
Micro-optimizations in Node.js Code
Micro-optimizations involve making small, focused improvements to parts of the code that are executed frequently or are otherwise critical to performance. While micro-optimizations should not be the first step in the optimization process, they can yield significant benefits once the larger bottlenecks are addressed.
For instance, consider a scenario where a function used to process user data is identified as a hot path through CPU profiling. One might optimize this function by:
- Unrolling loops to reduce the overhead of loop control structures.
- Replacing generic methods with more performant alternatives (e.g., using
Map
orSet
instead ofObject
for key-value storage). - Inlining small utility functions that are frequently called.
async_hooks
Event Loop Management with The async_hooks
module in Node.js provides an API to track asynchronous resources in the application, like promises or I/O operations. With async_hooks
, developers can gain visibility into the event loop and understand the state of asynchronous calls, which is crucial for identifying I/O bottlenecks.
Example use of async_hooks
to track asynchronous operations:
Documentation: Node.js Async Hooks
Worker Threads and Child Processes
Node.js is single-threaded by default, but CPU-bound tasks can be offloaded to separate threads or processes to avoid blocking the main thread and the event loop.
Using Worker Threads
The worker_threads
module allows you to run JavaScript in parallel, leveraging multiple CPU cores:
Workers can significantly improve the processing time of CPU-intensive tasks.
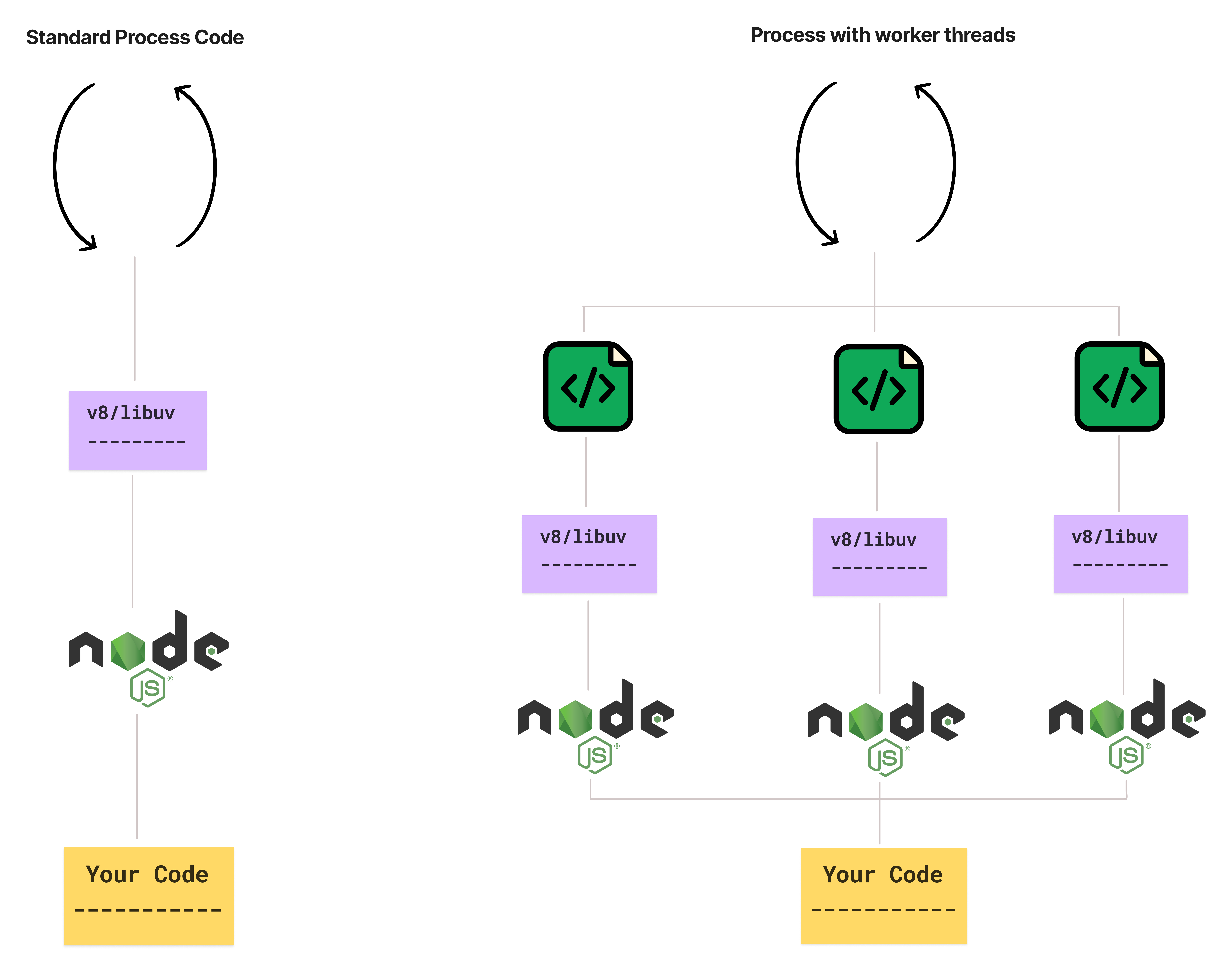
Documentation: Worker Threads in Node.js
Using Child Processes
For even more isolation, spawning child processes allows different parts of your Node.js application to run independently of each other:
By moving demanding workloads to child processes, the main event loop remains responsive for other operations.
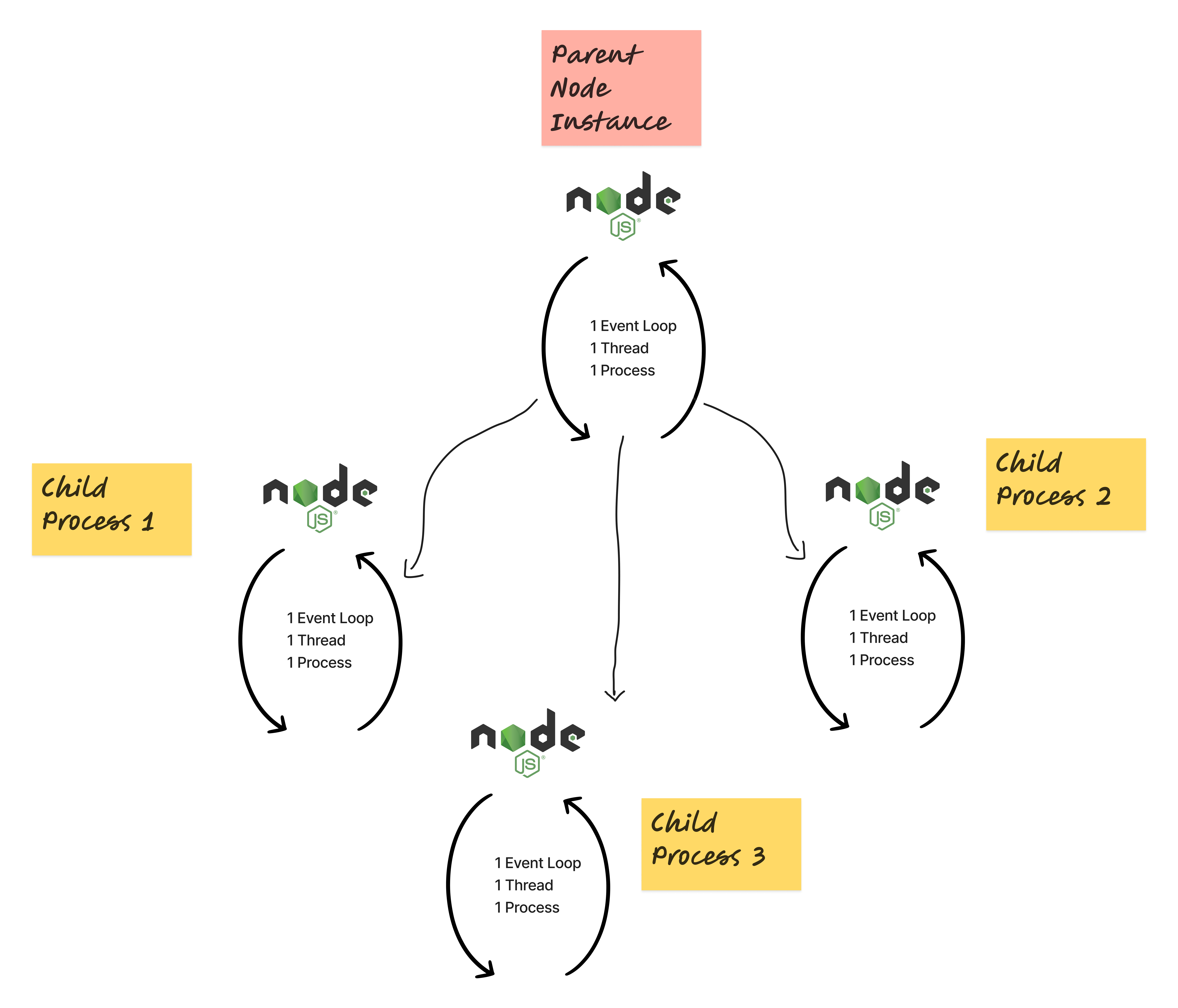
Documentation: Child Processes in Node.js
Advanced performance optimization in Node.js requires a keen understanding of your application's unique performance profile. Utilizing micro-optimizations, the async_hooks
module, worker threads, and child processes, developers can fine-tune their applications to achieve remarkable improvements in performance. It’s imperative, however, to profile and understand the impact of each optimization, as unnecessary changes can sometimes lead to maintainability issues without significant performance gains.
Best Practices for Sustained Performance
Maintaining superior performance in Node.js applications is not solely about identifying and fixing issues. It also involves establishing best practices that proactively prevent performance problems. Here are some strategies and tools to ensure your Node.js applications consistently perform well.
Coding Patterns and Practices
Choose the right algorithms and data structures for your use case, as they can have a significant impact on performance. For instance, prefer native methods over equivalent loops for array manipulation when readability and performance are concerns, and use asynchronous methods to avoid blocking the event loop.
Example of using native array methods:
Use Promises and async/await syntax to handle asynchronous code more intuitively, but be aware of potential pitfalls like the creation of unnecessary promise chains or the use of async functions in loops.
Streamlining Asynchronous Operations
When dealing with I/O, take advantage of Node.js's stream interface to handle data chunk by chunk without buffering it entirely in memory. This can prevent excessive memory use and keep your application responsive.
Example of using streams for file processing:
Regular Application Audits
Perform regular audits of your application's dependencies to find updates that might include performance improvements. Tools like npm's npm outdated
and npm audit
can help you keep your dependencies up to date and secure.
Regular Performance Profiling
Incorporate performance profiling into your development workflow. Tools like clinic.js and the built-in Node.js profiler can be used routinely to catch performance degradation early.
Automating Performance Optimization
Automate the integration of performance checks into your CI/CD pipeline. This can be achieved with tools like Lighthouse CI for web performance or GitHub Actions for automating workflows.
Example GitHub Action workflow for performance checks:
Monitoring and Management Tools
Utilize process managers like PM2 to keep your applications stable and make use of tools like Datadog, Grafana, Prometheus, or Sentry for real-time monitoring and error tracking.
Documentation and Tools:
- Node.js Best Practices
- NPM Documentation
- Clinic.js for Node.js
- Lighthouse CI
- GitHub Actions Documentation
By adhering to these best practices and routinely utilizing the relevant tools for code optimization and performance monitoring, Node.js developers can create high-performing applications that are resilient to production stresses and deliver a seamless experience to users.
Conclusion
Performance is a journey, not a destination. To ensure that your Node.js applications remain robust, responsive, and efficient, it's crucial to embed a culture of performance within your development teams. This involves not just reactive measures to tackle performance issues as they arise, but a proactive approach that integrates best practices and performance considerations at every stage of the application lifecycle.
The process begins with a solid understanding of the Node.js runtime environment and a commitment to writing optimized code. We've covered the necessity of real-time profiling and monitoring, using tools ranging from the built-in Node.js profiler to advanced solutions like clinic.js, Prometheus, and Grafana. We've delved into case studies that demonstrate the practical application of these tools to resolve actual performance bottlenecks, and we've explored advanced optimization techniques such as leveraging worker threads and child processes.
However, optimization is not without its trade-offs. While it's important to strive for peak application performance, one must also consider the maintainability and clarity of the code. Performance must be balanced against the cost of complexity that optimizations might add.
Moreover, we've emphasized the role of best practices, regular audits, and the automation of performance checks in maintaining sustained performance. With monitoring and error tracking services like Datadog and Sentry, developers can stay ahead of potential issues, addressing them before they impact the user experience.
In conclusion, a performant Node.js application is the result of intentional design, regular evaluation, and continual refinement. Maintain a proactive mindset, employ the right tools, and foster a performance-oriented culture to keep your Node.js applications running smoothly and efficiently. Remember, the tools and strategies are there to serve the greater goal of delivering an exceptional user experience, so leverage them to that end and not as an end in themselves.