Blog
Next.js next/image Under the Hood: SEO and Performance Optimization Secrets
Stay informed on new product features, the latest in technology, solutions, and updates.
Sep 2, 2023
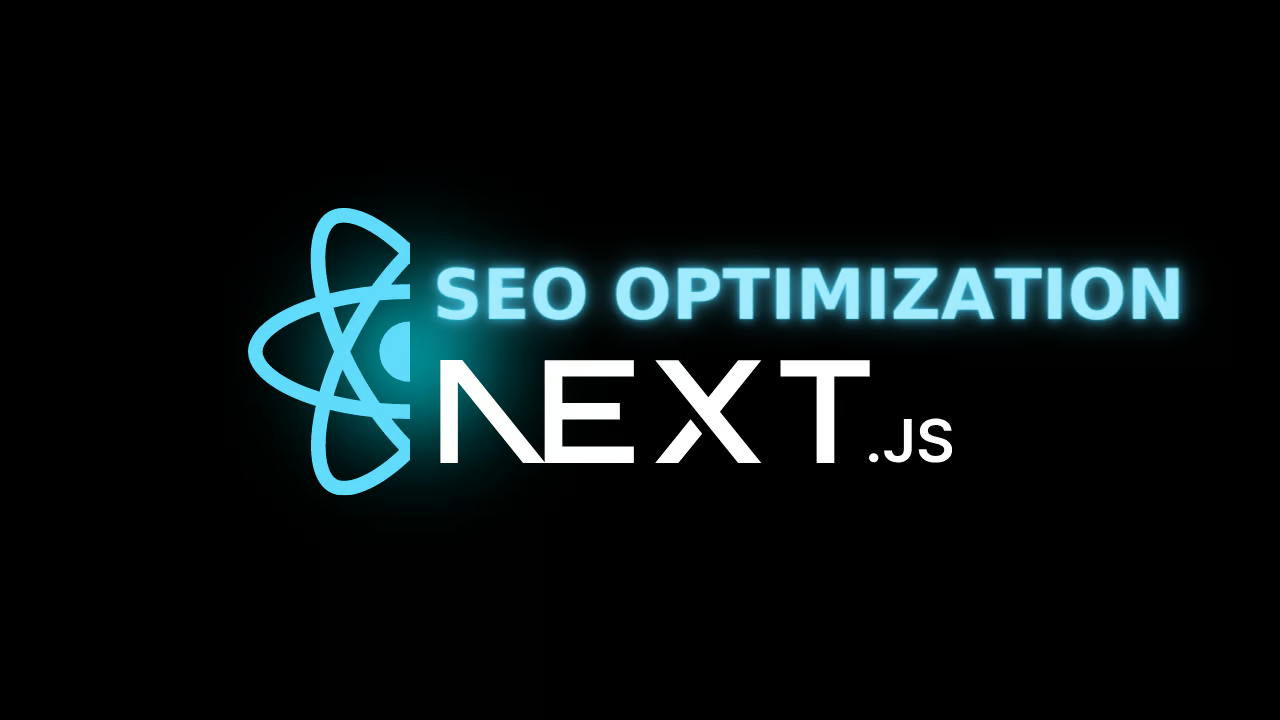
Introduction
In the fast-paced world of web development, performance and user experience often hinge on the efficiency of content delivery, with images playing a pivotal role. Enter the next/image
component from Next.js, a game-changer in how images are handled in modern web applications. This powerful feature is more than just an image tag—it's an out-of-the-box solution designed to automatically optimize your images for speed and performance.
But what magic lies behind this component? How does it effortlessly enhance the loading times, improve bandwidth usage, and ensure high-quality images across devices? In this blog, we peel back the layers of the next/image
component, exploring the intricate workings and sophisticated features that make it an indispensable tool for developers aiming to create fast, responsive, and visually stunning web applications.
From the intelligent loading techniques to the seamless integration with image hosting services, we'll dive deep into the mechanics that empower developers to deliver optimized images without the heavy lifting. Join us as we uncover the secrets behind the next/image
component, a cornerstone of Next.js that redefines image optimization in the era of modern web development.
1. Using Blur data to progressivly enhance image
One of the standout features of the next/image
component is its use of the Image Blur SVG technique, a strategy that significantly enhances the perceived performance of web applications. This ingenious approach not only captivates users with its aesthetic appeal but also cleverly masks the image loading time, creating a seamless user experience. But how exactly does this feature work under the hood?
The Mechanics Behind the Blur
When an image is called upon, the next/image
component doesn't simply begin to load the image in its final form. Instead, it first displays a lightweight, blurred version of the image, serving as a placeholder. This blurred version is quickly loaded, offering an immediate visual cue to users that content is on its way.
For Static Imported Images:
For images imported directly into a Next.js project, the process is beautifully automated. Upon encountering a static image, Next.js takes a snapshot of its essential attributes - such as dimensions - and utilizes the sharp
library to craft an optimized buffer. From this buffer, a small, blurred SVG is generated and temporarily displayed as the image loads in the background. This not only enhances the visual appeal during loading but also significantly reduces the amount of data transferred, ensuring a swift initial display.
As you can see in the code, we are just setting the placeholder as blur , this would automatically create a small blurred svg and temporarily display it before the image loads
For Extrenal Images
For images sourced from external URLs, the process adapts slightly. Developers have the flexibility to provide a custom blurDataURL
, which Next.js then uses as the placeholder. This ensures that even for images not stored within the project, the user experience remains consistently smooth and engaging.
We are specifically passing the blurDataURL, which is displayed while the actual image is being loaded
The Impact on User Experience
The use of a blurred image placeholder does more than just improve aesthetics; it fundamentally enhances the user's perception of loading times. In an era where attention spans are short, and patience is limited, this feature ensures that users remain engaged, even as the full-resolution image makes its way to their screen. Moreover, for users on slower connections, this technique provides a sense of immediate response, a critical factor in maintaining user engagement and satisfaction.
2. External Image Support
Next.js offers support for optimizing external images by providing simple configuration options. One of the key configuration requirements is specifying the hostname, while parameters like protocol, port, and path are optional. Here are relevant examples from the internet that demonstrate the features mentioned:
Size Variants:
Next.js automatically generates multiple versions of an image at different sizes to match the client's viewport and device pixel ratio. This ensures that the image is displayed with enhanced clarity on devices with varying screen resolutions. For example, if a user is viewing a webpage on a high-resolution device, Next.js would deliver a larger version of the image, while on a lower resolution device, a smaller version of the same image would be delivered. This helps to optimize the image display based on the device's capabilities.
Lazy Loading:
Next.js incorporates lazy loading techniques for images, where images are loaded only as they approach the viewport. This means that the images are fetched and downloaded by the browser only when they are about to be displayed on the user's screen. This approach conserves bandwidth and accelerates the rendering of the page. Lazy loading is especially useful for webpages with numerous images or large images. As an example, think of a long-scrolling webpage where images are only loaded as the user scrolls down the page, rather than all at once when the page loads initially.
Automatic Format Selection:
Upon receiving a request, Next.js conducts a browser check to ascertain support for contemporary image formats. In scenarios where a browser is compatible with advanced formats like WebP, Next.js opts to serve images in such formats to leverage their efficiency in compression and quality. Conversely, for browsers lacking this support, Next.js defaults to conventional formats such as JPEG or PNG. This dynamic format selection mechanism is instrumental in optimizing the delivery of images, tailoring the experience to the capabilities of the user's browser, thereby enhancing load times and visual fidelity.
Overall, Next.js provides valuable features for optimizing external images, such as generating image size variants, lazy loading, and automatic format selection. These features help improve webpage performance, conserve bandwidth, and enhance the visual experience for users.
Caching Mechanism
Optimized images are cached by Next.js, significantly reducing server load and facilitating faster content delivery. The caching strategy involves constructing unique filenames based on parameters such as maxAge
and etag
, followed by file system operations to manage the cached content effectively.
These technical details underscore the comprehensive approach Next.js adopts for image optimization, ensuring efficient, high-quality image delivery across diverse web environments.
3. Image Optimization with Sharp
Next.js leverages the sharp
library for advanced image optimization, including memory usage reduction, auto-detection of content types, and size detection. It also applies various optimizations like format-specific adjustments and orientation corrections, ensuring images are displayed correctly and efficiently.
Image optimization is a critical aspect of web development, especially when it comes to improving page load times and overall user experience. With Next.js, developers can take advantage of the powerful sharp library to optimize and enhance images in their applications.
Reducing Memory Usage
Next.js strategically limits the number of threads utilized by sharp, correlating to CPU cores, to minimize memory overhead. This is achieved through a calculated adjustment of sharp's concurrency settings, ensuring at least one thread remains active for image processing tasks. You can find the example from the official repo here
Content Type Auto-Detection
Next.js scrutinizes the initial bytes of image data to identify file signatures, enabling the classification of content types such as JPEG, PNG, and others. This preliminary step is crucial for determining the appropriate handling and optimization strategy for each image, this is done by reading the base64 data , find the example from official codebase here
Automatic Size Detection
For static images, Next.js utilizes the sharp package to extract metadata for AVIF images, and the imageSizeOf
function for JPEG, PNG, and WebP formats. This process involves decoding the image buffer to ascertain dimensions, ensuring images are accurately optimized for their intended display size.
Orientation and Parallel Processing
Next.js supports multiple orientations, adjusting images accordingly to maintain visual integrity. Furthermore, image operations are not performed sequentially; instead, they are compiled into an array and executed simultaneously, reducing processing time.
Advanced Optimization Techniques
Next.js applies several sophisticated optimization techniques to enhance image delivery:
- Orientation Correction: Utilizing EXIF data, images are rotated to their correct orientation before any resizing operations are performed.
- Aspect Ratio Maintenance: In the absence of specified dimensions, Next.js preserves the image's aspect ratio, ensuring visual consistency without unintended enlargement.
- Format-Specific Quality Adjustments: For non-AVIF images, Next.js processes images in the desired format with optimal quality settings. AVIF images undergo a quality reduction to conserve resources, alongside chroma subsampling to decrease color data size.
- Progressive JPEG Optimization: JPEG images are stored in a progressive format, improving the perceived load time by initially presenting a low-quality preview that gradually sharpens, enhancing user experience on slower connections.
Examples
Now, let's take a look at some code examples to demonstrate how to leverage Sharp in Next.js:
Basic image processing:
In this example, we import the sharp library and define a function processImage()
that takes an input path and an output path. We then use
Sharp's resize() method to resize the image to a specific width and height, and the toFile() method to save the processed image to the specified output path.
In this example, we resize the input image to a width of 300 pixels and a height of 200 pixels. The processed image is then saved as 'output.jpg'.
Automatic format detection:
In this example, we use Sharp's metadata() method to automatically detect the format of the input image.
In this example, the metadata() method returns an object that contains information about the image, including the format. The detected image format is then logged to the console.
These examples demonstrate just a few of the many ways you can leverage Sharp's powerful image processing capabilities in Next.js. By using Sharp, you can optimize and enhance the images in your applications, improving page load times and overall user experience.
4. Pixel Density Ratios
The component supports different pixel density ratios, generating images optimized for the device's screen resolution. This ensures high-quality visuals without unnecessary data consumption, although support for 3x pixel density ratios has been discontinued due to negligible benefits.
Pixel density ratios play a crucial role in optimizing images for different screen resolutions. With the advancement in technology, devices nowadays have varying pixel densities, which means the number of pixels per inch on the screen varies. To provide high-quality visuals without consuming unnecessary data, it is important to generate images that are optimized for the specific pixel density ratio of the device.
Pixel density is measured in pixels per inch (PPI) or dots per inch (DPI). The higher the pixel density, the crisper and more detailed the image will appear on the screen. For example, a device with a pixel density ratio of 2x will have twice as many pixels per inch compared to a device with a pixel density ratio of 1x.
To illustrate this further, let's consider an example. Suppose you have an image that is 100 pixels wide and 100 pixels tall. On a device with a pixel density ratio of 1x, this image will take up exactly the same amount of screen real estate (100 pixels by 100 pixels) when rendered. However, on a device with a pixel density ratio of 2x, the image will appear smaller (50 pixels by 50 pixels) but with higher clarity and detail.
To optimize images based on pixel density ratios, different versions of the same image can be created. Each version will have a specific width and height, determined by multiplying the original dimensions by the pixel density ratio. These optimized images can then be loaded dynamically based on the device's pixel density ratio, ensuring the best possible visual experience with minimal data consumption.
Consider the following code example in JavaScript, which demonstrates how to load different versions of an image based on the pixel density ratio:
In the above example, the window.devicePixelRatio
property is used to get the current pixel density ratio of the device. Depending on the value, the appropriate image URL is assigned to the imageUrl
variable. The image element is then created dynamically, and the src
attribute is set to the appropriate image URL. Finally, the image element is appended to the body
5. Integration with Image Hosting Services
Next.js seamlessly integrates with popular image hosting services like Akamai, Imgix, and Cloudinary, employing specific loaders to optimize images. These loaders adjust parameters such as format, size, and quality based on the image content and client capabilities.
For Akamai, Next.js constructs a URL with the desired height, width, and quality parameters to serve the optimized image.
With Imgix, Next.js sets the auto-parameter for auto-optimizations, along with width, fit, and quality parameters. It then constructs a URL and serves the image.
For Cloudinary, Next.js automatically selects the best file format based on the client's capabilities and image content. It also restricts the image's size to not exceed the given width and sets the quality. If the quality parameter is not provided, Next.js defaults to 'auto' and lets Cloudinary decide the optimal quality. Next.js constructs the URL using query parameters and serves the image.
These integrations ensure that images are optimized and delivered efficiently, enhancing the overall performance and user experience of your Next.js application.
References: