Blog
Discover and Optimize the Performance of your JavaScript Code
Stay informed on new product features, the latest in technology, solutions, and updates.
Jan 24, 2024
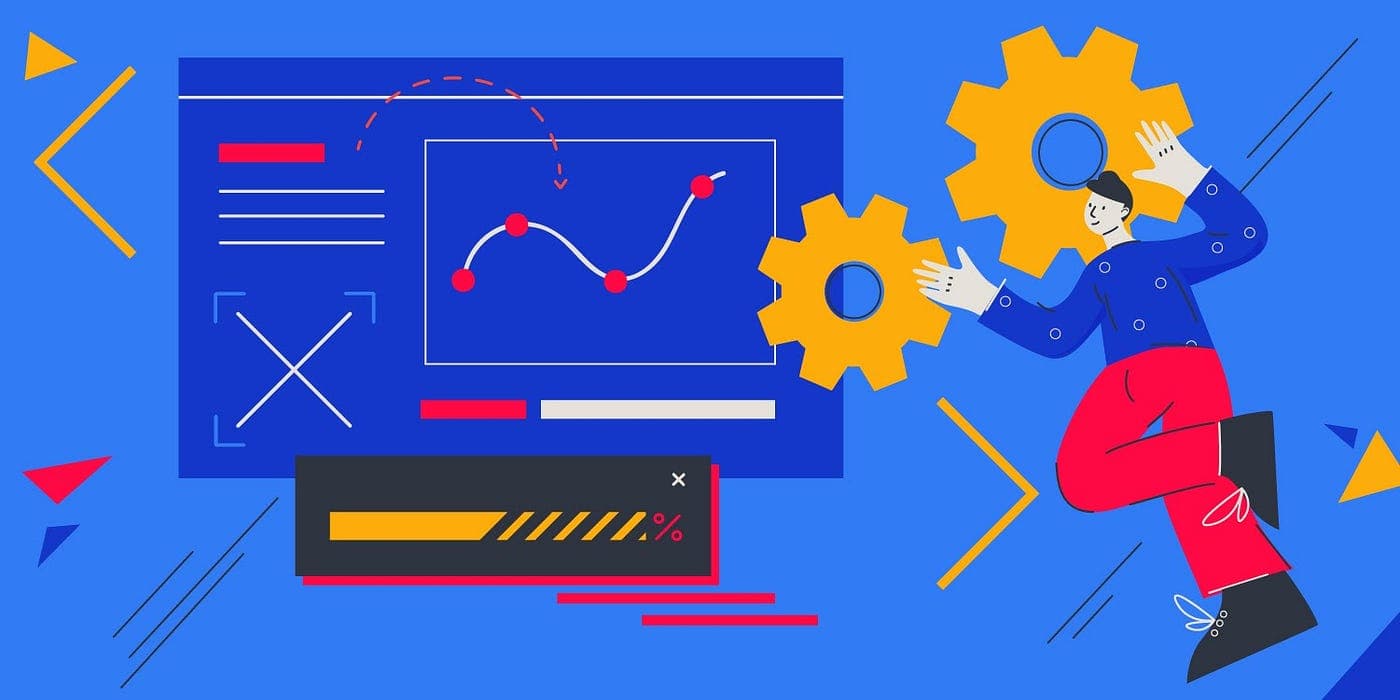
Introduction
In the world of web development, JavaScript plays a crucial role in creating interactive and dynamic websites. However, it can also become a performance bottleneck if not optimized properly. In this blog post, we will explore three effective ways to find and optimize the performance of your JavaScript code, helping you ensure a faster and smoother user experience.
In this blog post, we will explore effective ways to discover and optimize the performance of your JavaScript code. We'll dive into three key areas: ranking code by bytes, ranking code by scripting time, and ranking code by blocking time. By understanding and addressing these aspects, you'll be able to identify and improve the areas in your codebase that may be causing slowdowns and negatively impacting user experience.
By implementing the techniques and utilizing the tools discussed here, you'll be able to optimize the performance of your JavaScript code and ensure a faster, smoother, and more delightful user experience on your website.
Now, let's delve into the details and explore these performance optimization strategies for your JavaScript code.
1. Rank by Bytes: Lighthouse Treemap
One way to identify the worst JavaScript offenders is by analyzing their sizes using a tool like Lighthouse Treemap. This visual representation allows you to quickly identify the largest JavaScript files or modules in your codebase. Here's an example:
In this example, we have a JavaScript file called utility.js, which contains a function calculateSum that calculates the sum of two numbers and logs the result to the console. By using Lighthouse Treemap, you can visualize the sizes of JavaScript files in your codebase, and in this case, you will see the size of utility.js in the treemap representation. By identifying large files like this, you can focus on optimizing their size through techniques like minification, tree-shaking, and code splitting.
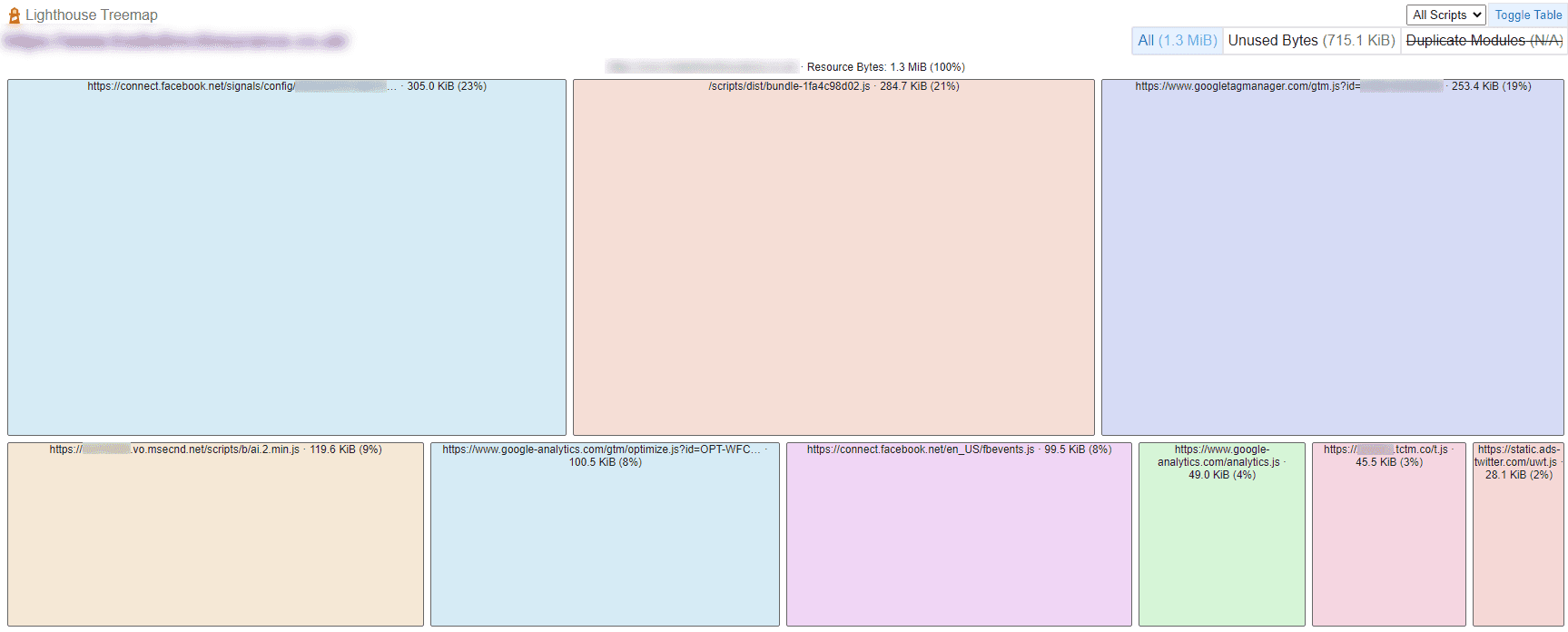
This visual representation allows you to quickly identify the largest JavaScript files or modules in your codebase and can prioritise optimization efforts by focusing on reducing the size of the largest files, improving overall performance. Here are some additional details:
- Lighthouse is a free, open-source tool from Google that audits the performance, accessibility, and other aspects of web pages.
- Lighthouse Treemap displays the file sizes of your JavaScript files as rectangles, allowing you to easily identify the largest files.
- By clicking on a rectangle, you can view additional details like file path, size breakdown, and other information.
To optimize the performance of your JavaScript code based on size, you can consider the following techniques:
- Minification: Reduces the size of your JavaScript files by removing unnecessary characters and whitespace.
- Tree-shaking: Removes unused code from your JavaScript files to reduce their size.
- Code splitting: Divides your JavaScript files into smaller, more manageable modules to reduce their size and improve caching.
2. Rank by Scripting Time: Dev Tools Performance Tab
Another crucial aspect of JavaScript performance optimization is monitoring the scripting time. The scripting time refers to the amount of time a script runs during page load. Using the Performance Tab in the Dev Tools, you can analyze the scripting time of each script. Here's an example:
In this example, we have a JavaScript function called performComputationallyIntensiveTask that performs a computationally intensive task using a loop. By using the Performance Tab in Dev Tools, you can analyze the scripting time of this function and identify it as a potential performance bottleneck. To optimize it, you can consider options like deferring the execution of this function until after the page has loaded, using techniques such as requestAnimationFrame() or setTimeout(). This allows the page to render faster and be more responsive to user interactions.
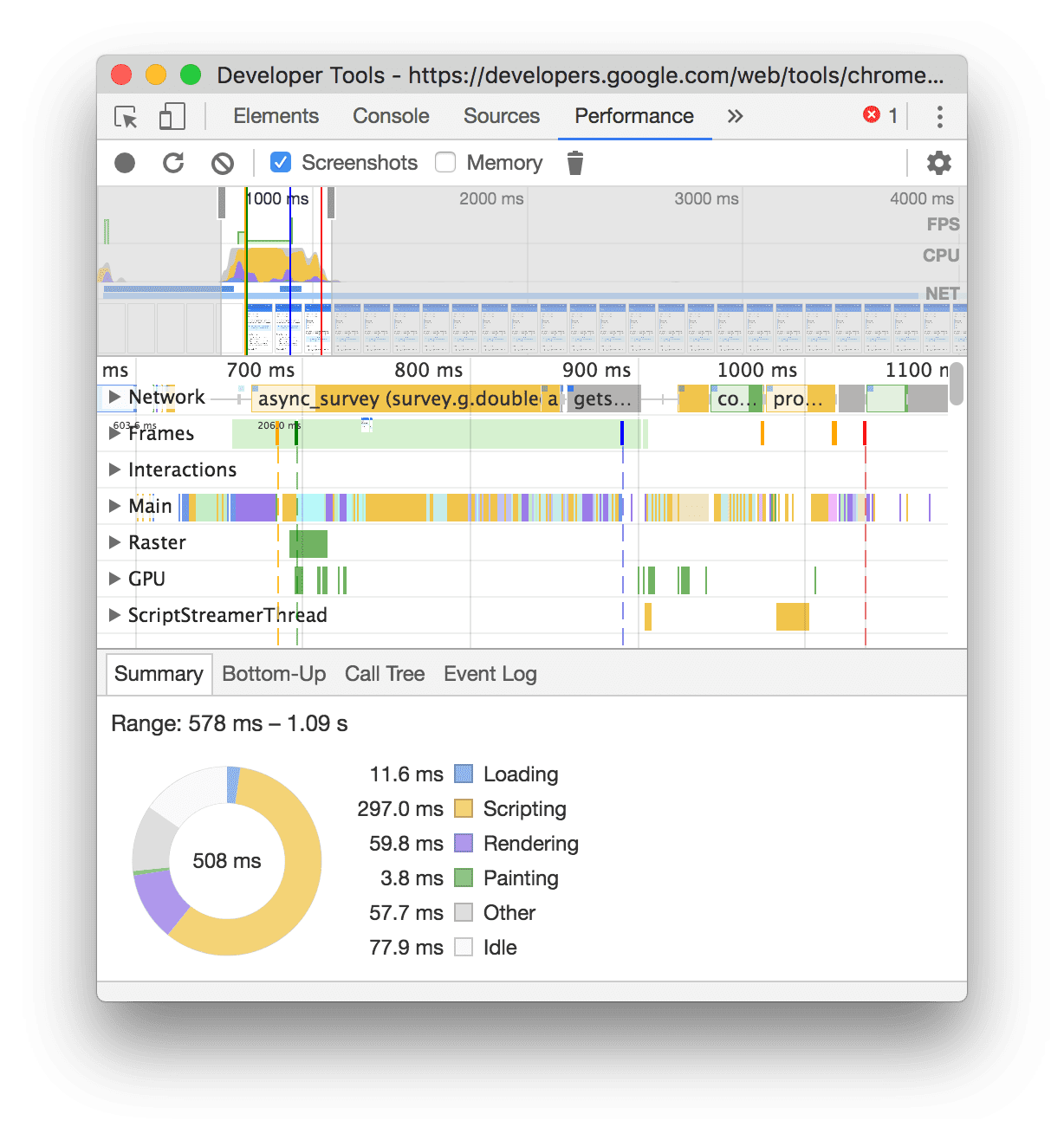
By analyzing the scripting time, you can identify scripts that require excessive processing and optimize them to reduce execution time, improving page load performance. Here are some additional details:
- The Dev Tools is a powerful set of debugging and performance analysis tools built into most modern web browsers, including Chrome, Firefox, and Safari.
- The Performance Tab displays a timeline of the events that occur during page load, including the scripting time of each script.
- You can use the filtering and sorting options in the Performance Tab to identify scripts that take a long time to execute.
To optimize the performance of your JavaScript code based on scripting time, you can consider the following techniques:
- Use requestAnimationFrame() or setTimeout() to defer non-essential JavaScript code until after the page has loaded.
- Avoid running computationally-intensive JavaScript code on the main thread, which can cause performance bottlenecks.
- Use Web Workers or web APIs like the Background Fetch API to perform background tasks and improve performance.
3. Rank by Blocking Time: WebPageTest
Blocking time is a critical metric that can significantly impact user experience. It measures the amount of time the main thread is blocked, preventing the browser from responding to user input. Utilising tools like WebPageTest, you can rank script origins based on their total blocking time. Here's an example:
In this example, we have a JavaScript code snippet that blocks the main thread during the DOMContentLoaded event. This can negatively impact the user experience as it prevents the browser from responding to user input promptly. By using WebPageTest and analyzing the waterfall chart, you can identify scripts that cause high blocking time. For scripts like the above example, you can optimize by deferring their execution until after the page has loaded, using techniques like async or defer attributes in script tags. This allows the page to load faster and become interactive sooner.
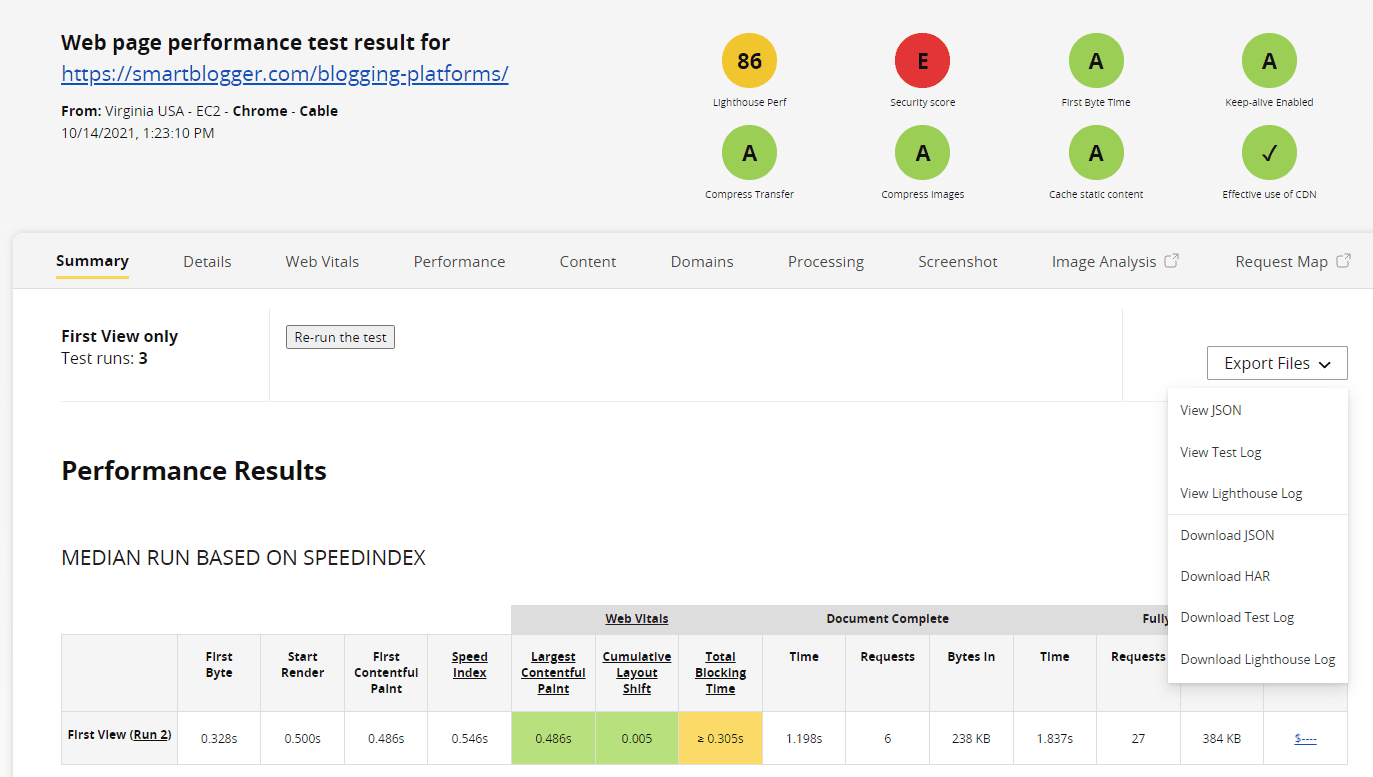
By identifying scripts that cause high blocking time, you can optimize their execution to minimize the impact on user interaction, resulting in a more responsive website. Here are some additional details:
- WebPageTest is a free online tool that allows you to test the performance of your website from various locations and devices.
- The tool provides detailed reports on various performance metrics, including blocking time for scripts.
- You can use the waterfall chart in WebPageTest to identify scripts that cause high blocking time.
To optimize the performance of your JavaScript code based on blocking time, you can consider the following techniques:
- Use the async and defer attributes in your script tags to improve loading performance and reduce blocking time.
- Optimize the critical rendering path of your website by prioritizing essential scripts and stylesheets.
- Use lazy loading techniques to defer the loading of non-essential scripts until after the page has loaded, reducing blocking time.
Conclusion
Optimising the performance of your JavaScript code is crucial for delivering a fast and efficient user experience. By utilizing techniques like ranking by bytes, scripting time, and blocking time, you can identify and optimize the worst JavaScript offenders in your codebase. Remember to regularly review and optimize your JavaScript code to ensure optimal performance and improve user satisfaction. Remember, improving JavaScript performance is an ongoing process, and there are numerous other techniques and best practices to explore. Stay tuned for more in-depth articles on optimising First Input Delay and Interaction to Next Paint in a future blog post. Some additional techniques you can consider include:
- Using a Content Delivery Network (CDN) to improve loading speed and reduce server load.
- Using static site generators like Gatsby or Next.js to create websites with excellent performance and SEO.
- Using browser caching and compression to reduce server requests and improve loading speed.
We hope you found this blog post helpful! If you have any questions or would like to share your own JavaScript performance optimization tips, feel free to contact us.