Blog
Building High-Performing Next.js Apps with GraphQL
Stay informed on new product features, the latest in technology, solutions, and updates.
Sep 2, 2023
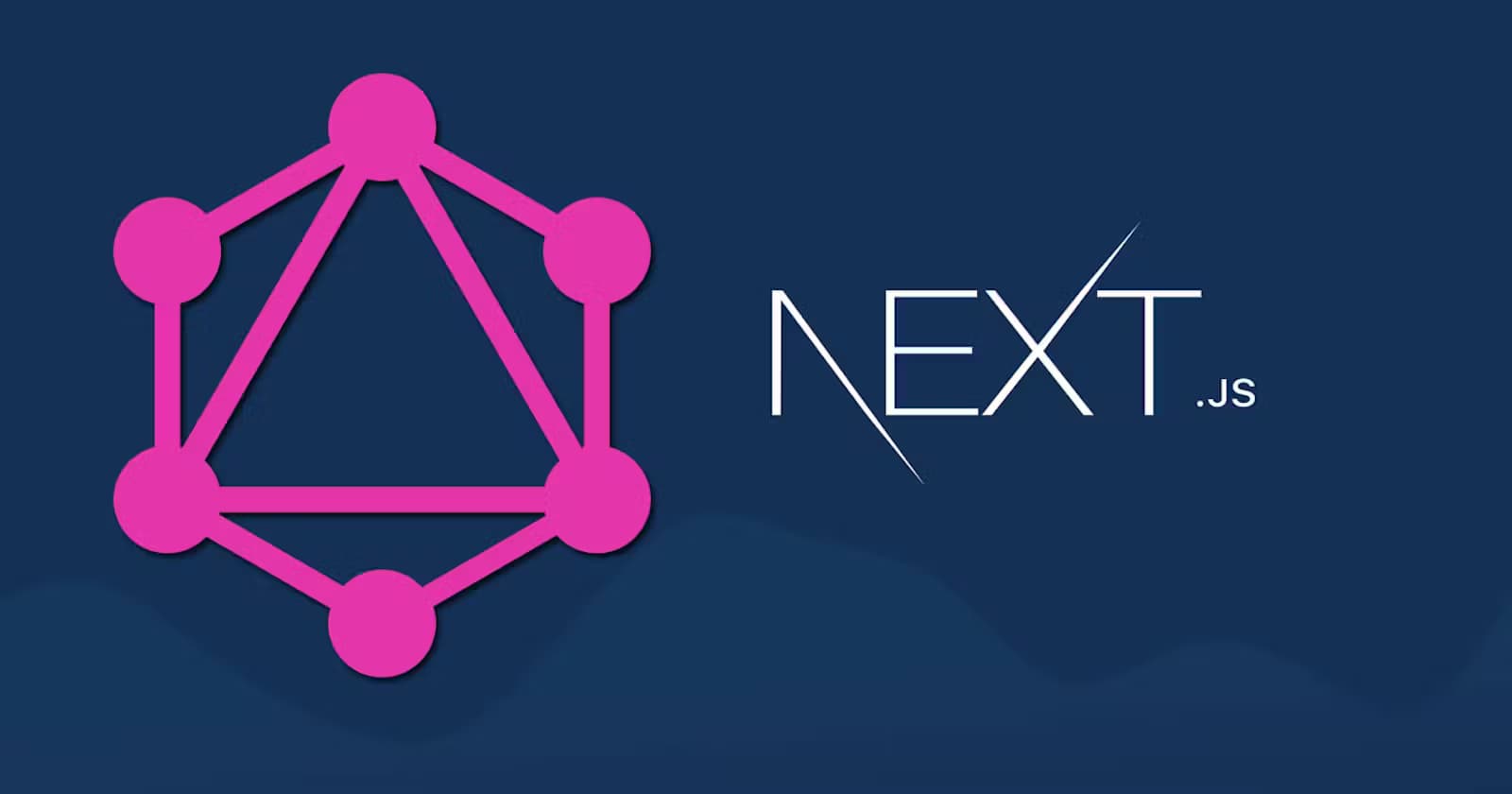
Introduction
GraphQL is a query language that allows you to efficiently fetch data from APIs. It provides a flexible and efficient way to retrieve only the data you need, reducing the amount of unnecessary data transfer. Next.js is a popular React framework for building server-side rendered and statically generated websites. It combines the benefits of server-side rendering with the ease of use of React components. By combining GraphQL with Next.js, you can create powerful and efficient applications that efficiently fetch and manage data.
Step 1: Setting up a Next.js project
To set up a Next.js project, first make sure you have Node.js installed on your machine. Then, open your terminal and run the following commands:
- Run
npx create-next-app my-app
to create a new Next.js project named "my-app". This command will set up a basic Next.js project structure for you. - Navigate into the project folder by running
cd my-app
.
Step 2: Adding GraphQL to the project
To use GraphQL in your Next.js project, you need to install the necessary packages. In the terminal, run the following command:
- Run
npm install @apollo/client graphql
to install the Apollo Client and GraphQL packages. Apollo Client is a powerful GraphQL client that integrates seamlessly with Next.js, and graphql is the official JavaScript implementation of the GraphQL specification.
Step 3: Creating a GraphQL schema
A GraphQL schema defines the types of data available in your API and the operations that can be performed on that data. To create a GraphQL schema, follow these steps:
- Create a new file called
schema.graphql
in the root folder of your Next.js project. - Inside the
schema.graphql
file, define your GraphQL schema using the GraphQL Schema Definition Language (SDL). The SDL allows you to define the structure of your data types, their attributes, and the relationships between them. - Here's an example of a simple schema that defines a
User
type withid
,name
, andemail
fields:
Step 4: Implementing GraphQL resolvers
In resolvers.js, you can define the resolver functions for each field in your schema. Here's an updated version of the resolvers file that includes implementations for the new types:
Step 5: Connecting to a GraphQL server
To connect a Next.js app to a separate GraphQL server, you need to update the apolloClient.js file or use environment variables to configure the server URL. Here's how you can do it:
- Update the apolloClient.js file:
- Open the apolloClient.js file in your Next.js project.
- Look for the section where the Apollo Client is being initialized.
- Find the uri or link property and update it with the URL of your GraphQL server.
- Save the file.
Example:
By following these instructions, you will successfully connect your Next.js app to the GraphQL server. This allows you to fetch and mutate data from the server using GraphQL queries and mutations in your Next.js components.
Step 6: Fetching data in Next.js pages
In a Next.js page (e.g., pages/users.js), you can fetch and display data from the GraphQL server using the useQuery hook provided by Apollo Client. Here's an example of fetching and rendering a list of users:
Step 7: Caching and local state management
Caching is an essential feature in Apollo Client that allows for efficient management of data fetched from a GraphQL server. When a query is made, Apollo Client first checks its cache to see if the required data is already available. If the data is present in the cache, it is returned immediately, eliminating the need for a network request.
Apollo Client's cache is a normalized data store which organizes data into a graph-like structure. Each piece of data is identified by a unique key, making it easy to retrieve and update specific items. By default, Apollo Client normalizes and stores the data based on the schema defined by the GraphQL server.
Here's an updated example using the same user delete functionality with local state management in Next.js:
In this example, we have integrated the user delete functionality using Apollo Client's useMutation
hook. When the delete button is clicked, the handleDeleteUser
function is triggered. Inside this function, the deleteUser
mutation is executed and the cache is manually updated using the update
function provided by Apollo Client. The update
function reads the existing users from the cache, filters out the user that was deleted, and writes the updated list of users back to the cache using cache.writeQuery
. This ensures that the UI reflects the deletion of the user without needing to make another network request.
Additionally, we have added handling for the delete loading state and error state. If the delete operation is in progress, a loading message is displayed. If there is an error during the delete operation, an error message is shown.!
Conclusion and Next Steps
In this article, we walked through the process of integrating GraphQL into a Next.js application. We discussed the benefits of using GraphQL and how it can improve the efficiency of data fetching by allowing clients to request only the data they need. We also explored how to set up a GraphQL server with Apollo Server and connect it to a Next.js application using Apollo Client.
Throughout the article, we used an example of a user management system to demonstrate the implementation of GraphQL in Next.js. We covered various steps, including setting up the server, defining the GraphQL schema and resolvers, creating queries and mutations, and connecting the client to the server in the Next.js application. We also explored concepts like pagination and local state management with Apollo Client.
By following these steps, you should have a solid understanding of how to integrate GraphQL into your Next.js projects and leverage the power of GraphQL to efficiently fetch and manipulate data.
To further explore GraphQL with Next.js, here are some additional resources:
- Apollo GraphQL documentation - The official documentation for Apollo GraphQL provides comprehensive guides, tutorials, and references for both the server and client implementations: Apollo GraphQL Docs
- Next.js documentation - The official documentation for Next.js covers various topics, including data fetching methods, API routes, and server-side rendering: Next.js Docs
- GraphQL tutorials on Next.js - Next.js has a dedicated section on the official tutorial website that covers topics such as GraphQL, data fetching, and server-side rendering: Next.js Tutorials
- GraphQL tutorial with Apollo Client - Apollo Client has a tutorial that covers using Apollo Client with GraphQL in a React application, which can be adapted to a Next.js project: Apollo Client Tutorial
- GraphQL subscriptions and real-time updates - To explore real-time updates and subscriptions with GraphQL and Next.js, you can refer to the Apollo documentation on this topic: GraphQL Subscriptions with Apollo
I encourage you to start experimenting with GraphQL in your own Next.js projects. You can leverage the power of GraphQL to optimize data fetching, manage local state, and build efficient and flexible APIs.
I hope this article and the provided examples have helped clarify the implementation process. If you have any further questions, need more specific examples, or require additional assistance, feel free to contact us.